Security control validation and enforcement are tasks we have regularly. One of those never-ending efforts is to ensure the right combination of protocols, ciphers, and algorithms are available. Security experts have tools and methods we don't have and must develop substitutes.
Cipher sites have my special love since they have different naming conventions in different frameworks or products. The OpenSSL community even keeps the list of cipher suite name mappings as a part of the product documentation. Through the years, I've created numerous connectivity validators with Java or Shell. But every time, I had to identify ciphers from the report, translate them to the "native" format, and then interpret the results, arguing that the ciphers in my output were the same as the original.
Here is yet another secure socket validation utility written in Python. Due to Python's data-processing capabilities, you can write code that requires much more effort in other languages. The small application in this repository can test secure listeners with a subset of ciphers in any convention from the OpenSSL documentation page. In essence, the script performs the steps as follows:
- Load cipher suite names into a list of tuples.
In [11]: cipher_names[-10:]
Out[11]:
[('TLS_ECDHE_PSK_WITH_CHACHA20_POLY1305_SHA256',
'ECDHE-PSK-CHACHA20-POLY1305'),
('TLS_DHE_PSK_WITH_CHACHA20_POLY1305_SHA256',
'DHE-PSK-CHACHA20-POLY1305'),
('TLS_RSA_PSK_WITH_CHACHA20_POLY1305_SHA256',
'RSA-PSK-CHACHA20-POLY1305'),
('TLS_AES_128_GCM_SHA256',
'TLS_AES_128_GCM_SHA256'),
('TLS_AES_256_GCM_SHA384',
'TLS_AES_256_GCM_SHA384'),
('TLS_CHACHA20_POLY1305_SHA256',
'TLS_CHACHA20_POLY1305_SHA256'),
('TLS_AES_128_CCM_SHA256', 'TLS_AES_128_CCM_SHA256'),
('TLS_AES_128_CCM_8_SHA256', 'TLS_AES_128_CCM_8_SHA256'),
('SSL_DHE_RSA_WITH_3DES_EDE_CBC_SHA', 'EDH-RSA-DES-CBC3-SHA'),
('SSL_DHE_DSS_WITH_3DES_EDE_CBC_SHA', 'EDH-DSS-DES-CBC3-SHA')]
In [12]:
The last ten cipher name pairs
- Search through the ciphers to find any matches
#Walk through the list of incoming ciphers
for cp in test_ciphers:
# Find matching name tuple in a list
ctpl = [item for item in cp_list if cp in item][0]
Find matching cipher name pairs in both conventions.
- Tests connection against internet address using matching cipher suite name.
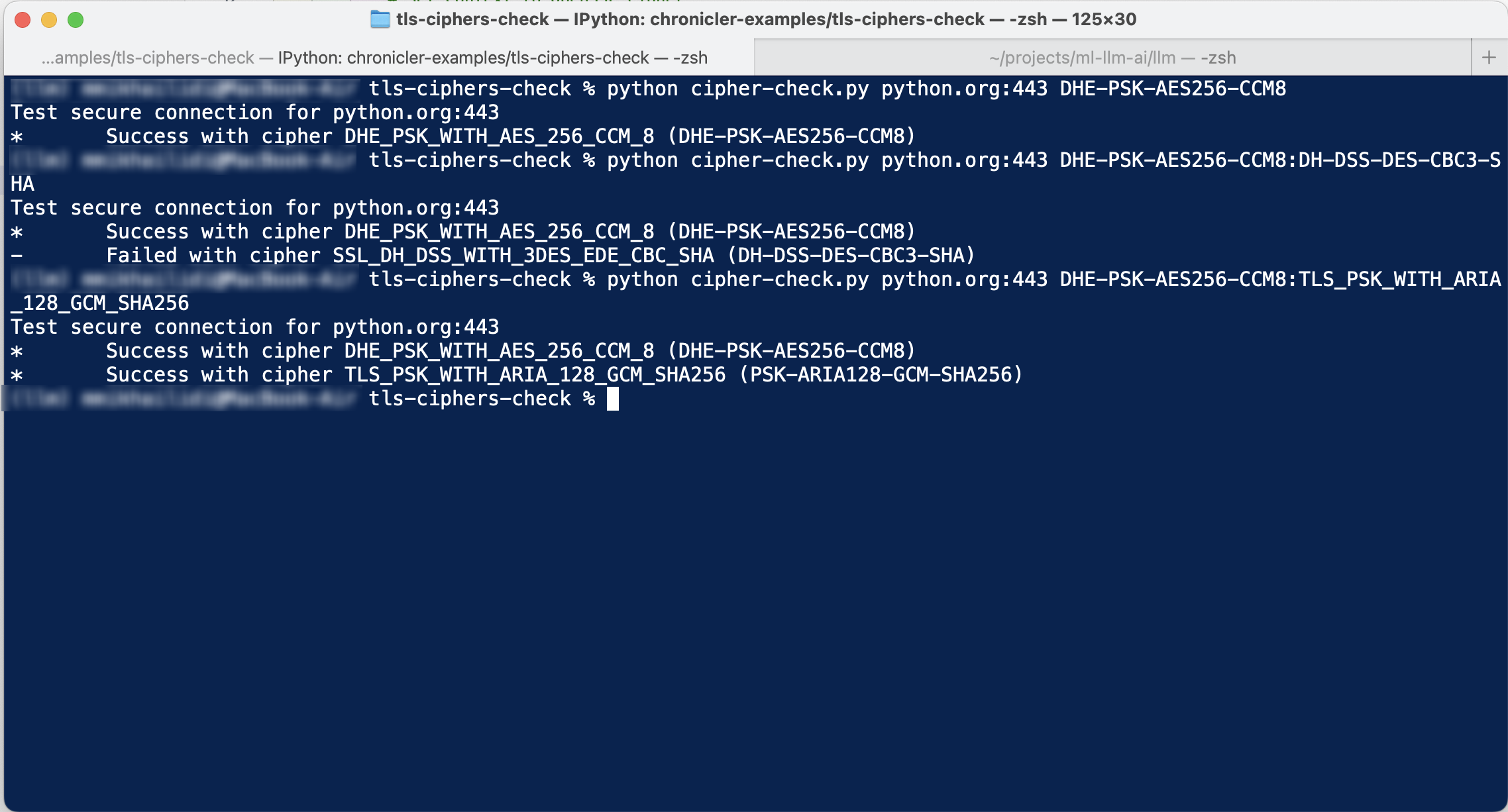
The code intentionally doesn't use advanced modules to make it as portable and compatible as possible.