Recently I ran into a problem with an Oracle WebLogic Server data source. We were getting an IO Error: Connection reset
in the console and logs when we try to start up the data source.
I could connect to the target database using a client tool such as Oracle SQL Developer, which indicated that the problem was likely not on the database server. So I downloaded the SQL*Plus Instant Client onto my server, and I was able to successfully connect using sqlplus as well.
This seemed to indicate that the problem was not the network or the database, but possibly something with the JDBC driver.
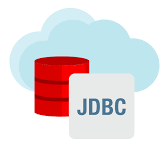
So how can you test the JDBC driver from WebLogic without having to write some Java code? Fortunately, there is a utility called utils.dbping
which you can use for this exact purpose. To execute it is simple:
cd $DOMAIN_HOME/bin
. setDomainEnv.sh
java utils.dbping ORACLE_THIN dbuser dbpassword dbhost:1521/dbsid
Here is an example of a successful connection:
oracle@soahost:/u01/app/oracle/domains/soa_domain> java utils.dbping ORACLE_THIN dbuser dbpassword dbhost:1521/dbsid
**** Success!!! ****
You can connect to the database in your app using:
java.util.Properties props = new java.util.Properties();
props.put("user", "dbuser");
props.put("password", "********");
java.sql.Driver d =
Class.forName("oracle.jdbc.OracleDriver").newInstance();
java.sql.Connection conn =
Driver.connect("dbuser", props);
Here is an example of an unsuccessful connection:
oracle@soahost:/u01/app/oracle/domains/soa_domain> java utils.dbping ORACLE_THIN dbuser dbpassword dbhost:1521/dbsid
Error encountered:
java.security.PrivilegedActionException: java.sql.SQLRecoverableException: IO Error: Connection reset
at java.security.AccessController.doPrivileged(Native Method)
at utils.dbping.getConnection(dbping.java:322)
at utils.dbping.main(dbping.java:287)
Caused by: java.sql.SQLRecoverableException: IO Error: Connection reset
at oracle.jdbc.driver.T4CConnection.logon(T4CConnection.java:816)
at oracle.jdbc.driver.PhysicalConnection.connect(PhysicalConnection.java:793)
at oracle.jdbc.driver.T4CDriverExtension.getConnection(T4CDriverExtension.java:33)
at oracle.jdbc.driver.OracleDriver.connect(OracleDriver.java:614)
at java.sql.DriverManager.getConnection(DriverManager.java:664)
at java.sql.DriverManager.getConnection(DriverManager.java:208)
at utils.dbping$1.run(dbping.java:327)
... 3 more
Caused by: java.net.SocketException: Connection reset
at java.net.SocketInputStream.read(SocketInputStream.java:209)
at java.net.SocketInputStream.read(SocketInputStream.java:141)
at oracle.net.nt.MetricsEnabledInputStream.read(TcpNTAdapter.java:759)
at oracle.net.ns.Packet.receive(Packet.java:312)
at oracle.net.ns.DataPacket.receive(DataPacket.java:106)
at oracle.net.ns.NetInputStream.getNextPacket(NetInputStream.java:306)
at oracle.net.ns.NetInputStream.read(NetInputStream.java:250)
at oracle.net.ns.NetInputStream.read(NetInputStream.java:172)
at oracle.net.ns.NetInputStream.read(NetInputStream.java:90)
at oracle.jdbc.driver.T4CSocketInputStreamWrapper.readNextPacket(T4CSocketInputStreamWrapper.java:124)
at oracle.jdbc.driver.T4CSocketInputStreamWrapper.read(T4CSocketInputStreamWrapper.java:80)
at oracle.jdbc.driver.T4CMAREngineStream.unmarshalUB1(T4CMAREngineStream.java:452)
at oracle.jdbc.driver.T4C8TTIdty.receive(T4C8TTIdty.java:711)
at oracle.jdbc.driver.T4C8TTIdty.doRPC(T4C8TTIdty.java:616)
at oracle.jdbc.driver.T4CConnection.connect(T4CConnection.java:1798)
at oracle.jdbc.driver.T4CConnection.logon(T4CConnection.java:539)
... 9 more
This utility is incredibly useful because it uses the same exact JDBC driver that is configured in your WebLogic instance.
In our particular problem, we were able to confirm that the issue was related to the MTU size at the network adapter level:
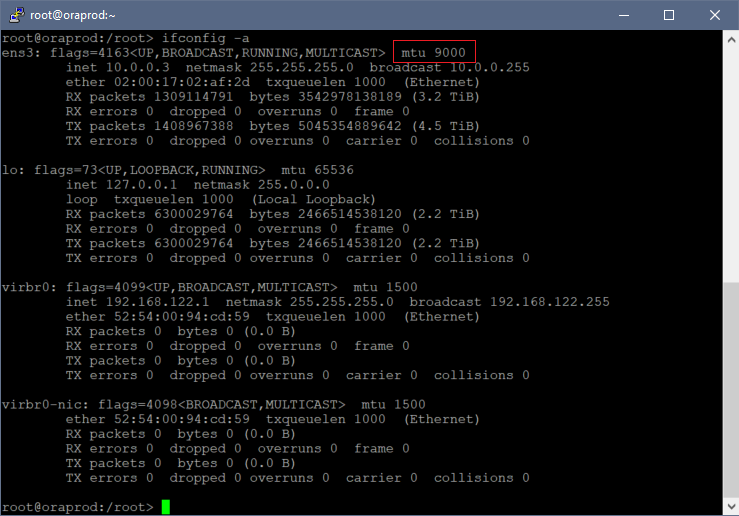