Automate Housekeeping
To be honest, my house is not the brightest kid on the block. Of course, we have Roomba, some smart light switches, a few IP cameras, an advanced mesh network, and a couple Alexa stations. Yet all of it is not connected or doesn't work the way I'd like to. The main reason for such a hectic approach is I'm a lazy person. That's and of course money. For one who does automation for a living, it's okay to be a bit lazy. So let's talk about how I keep my local git repositories in order with minimal effort.
From time to time, I post items around Ansible, Ansible Tower, and GitLab repositories. So, you may see that it consumes a noticeable amount of my work time. I ranted about GitLab repositories, projects, inventories, and hybrid configurations a lot. So, it's time to take a look at my workstation.
I do Oracle Fusion Middleware automation, yet I rarely use Oracle development tools. Instead, I spend my days with Microsoft VS Code, Git, and SSH terminal. I don't want to tell you how great VS Code is or some tips and tricks around Git; I'd love to learn more myself. Yet you may have similar struggles, managing local repositories.
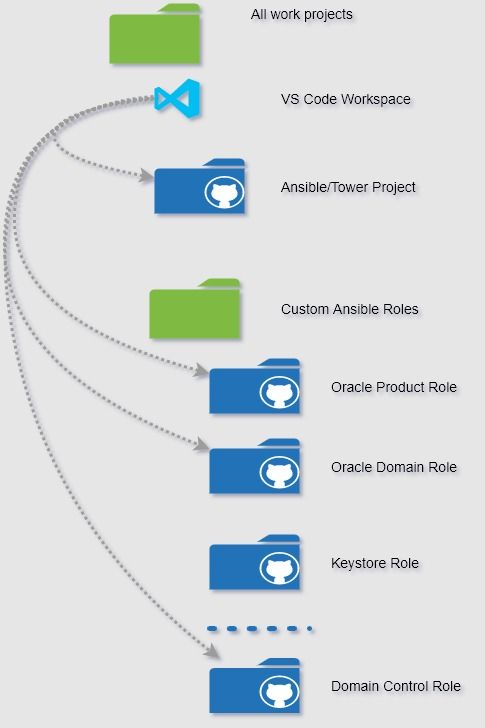
The simplest VS Code workspace I can imagine uses at least two Git/GitLab repositories:
- Project repository
- Inventory, through the submodule reference
Projects with custom roles consume significantly more than two repositories, and as a consequence VS Code struggles with multiple Git repositories within the project. It's painful to perform multiple commits or updates all local repositories. With the score of roles, even the simple repository clone turns to a project and begs for automation, if you ask me.
After the third project clone, I gave up and created a small shell script to run git commands against multiple local repositories. It has grown from a single clone command and expanded functionality with time. This one is the most recent version, cleaned from the project-specific details.
#!/bin/sh
##############################
# Michel Mikhailidi
# mm@chronicler.tech
# August, 2020
##############################
# List your role names here.
# I use role name as a repository name
ROLES=( "certificate" "domain-control" "weblogic-install" \
"product-install" "product-patch" "weblogic-patch" "rcu" "keystore")
# Role base is the location of all your local repositories
# Script uses it for all repository operations
# Example below represents Windows 10 mounts in the Shell console
#ROLES_BASE=/c/my-projects/ansible-roles
ROLES_BASE=$(pwd)
# All role projects are in the same group on SCM server
# U can use SSH or HTTPS links for the groups reference.
ROLES_GROUP=https://github.com//my/ansible/tower/roles
# The first parameter specify the operation
# I have implemented: clone, pull, push, checkout, and status.
ops=$1
# Default branch name is master
brnch=${2:-master}
case $ops in
clone)
cd $ROLES_BASE
for rl in ${ROLES[@]}; do
git clone ${ROLES_GROUP}/${rl}.git
done
;;
checkout)
for rl in ${ROLES[@]}; do
echo -e "Push for ${rl} \
\n=========================================\n"
cd $ROLES_BASE/$rl
git $ops $brnch
git pull origin $brnch
done
;;
pull)
for rl in ${ROLES[@]}; do
echo -e "Push for ${rl} \
\n=========================================\n"
cd $ROLES_BASE/$rl
git pull origin $brnch
done
;;
push)
for rl in ${ROLES[@]}; do
echo -e "Push for ${rl} \
\n=========================================\n"
cd $ROLES_BASE/$rl
git $ops origin $brnch
done
;;
status)
for rl in ${ROLES[@]}; do
echo -e "Push for ${rl} \
\n=========================================\n"
cd $ROLES_BASE/$rl
git $ops
done
;;
*)
echo "Operation git ${ops} ${brnch} is not yet implemented."
;;
esac
You may never need such a thing, but if you want to use it - don't forget to customize the code:
- ROLES - Array of the project names to work with. I left only a fraction of my original list;
- ROLES_BASE - Path to the folder where you keep your local projects. The exact path format depends on what Shell interpreter you use.
- ROLES_GROUP - Your Git server URL. In my case, all repositories are under the same group. You may never need them if you have all repositories cloned already.
With this script, I can manipulate the local repositories much faster and more effectively. There are some examples:
# Prepare new roles folder
[user@workstation]$ mkdir -p /c/my-projects/new-roles
[user@workstation]$ cd /c/my-projects/new-roles
# Initiate Local repostories
[user@workstation]$ mass-git-ops.sh clone
# Checkout the same branch
[user@workstation]$ mass-git-ops.sh checkout dev-branch
# Check the statatus of local repositories
[user@workstation]$ mass-git-ops.sh status